How to Integrate Twilio with Rails App to Send SMS
Follow our step-by-step guide for seamless Twilio integration with Rails for sending SMS.
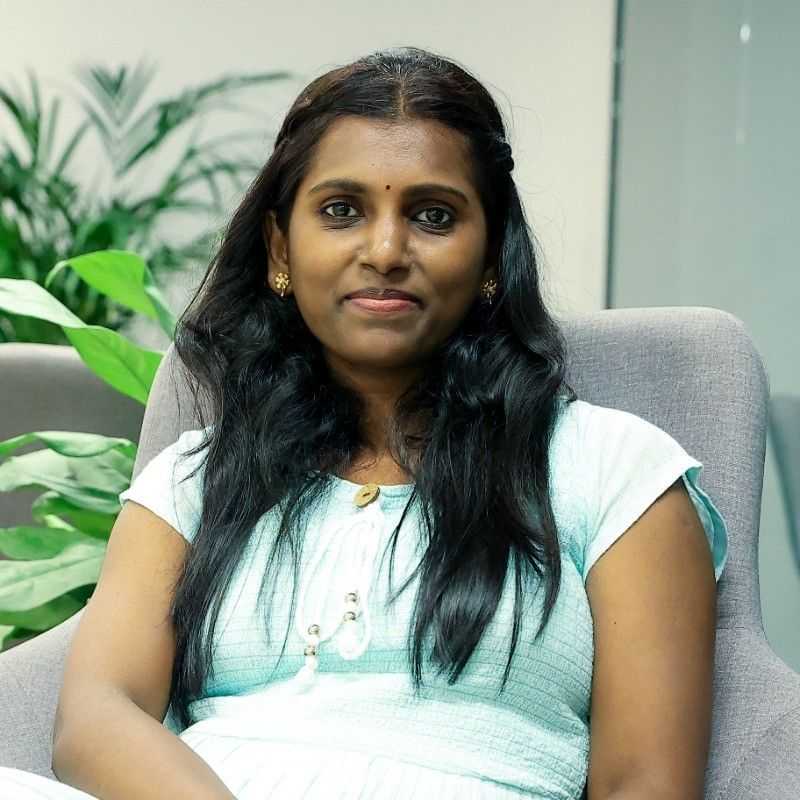
Raisa Kanagaraj
Technical Content Writer
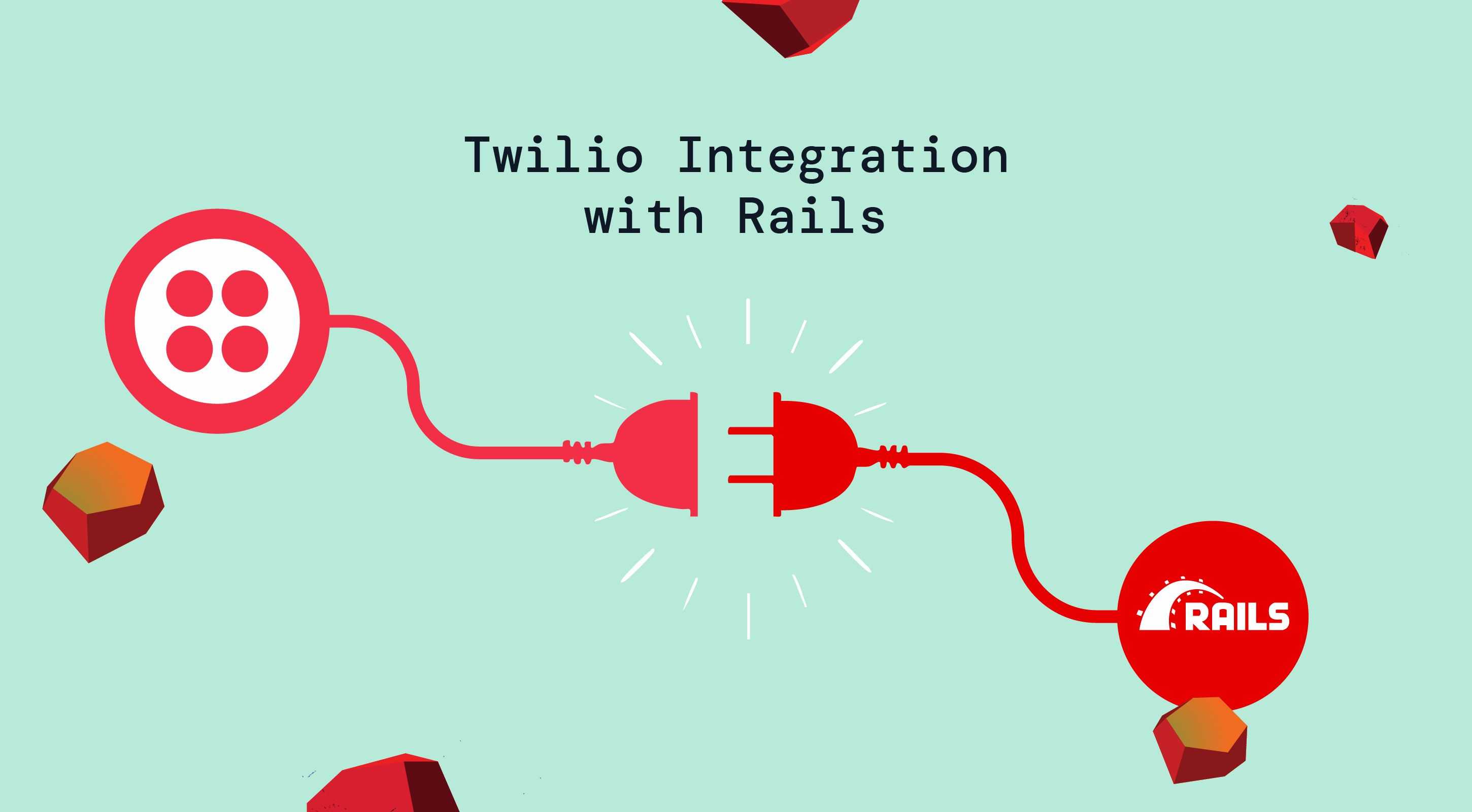
Integrating Twilio with a Ruby on Rails application enables developers to send SMS notifications programmatically. Whether you're building a user authentication system, appointment reminders, or marketing campaigns, Twilio simplifies SMS delivery with its robust API.
In this guide, we’ll walk through setting up Twilio integration with Rails app to send SMS messages step by step.
Prerequisites
Before starting, ensure you have the following:
- A Ruby on Rails application (Rails 6 or later recommended)
- A Twilio account (Sign up for free)
- Installed Twilio Ruby SDK
- Your Twilio credentials (Account SID, Auth Token, and Twilio Phone Number)
Step 1: Set Up Your Twilio Account
- After signing up, navigate to the Twilio Console.
- Note your Account SID and Auth Token (under "Account Info").
- Purchase a Twilio phone number (via "Phone Numbers" > "Buy a Number"). Trial accounts can send SMS only to verified numbers.
If you're unsure about managing these configurations, consider hiring experienced Ruby on Rails developers.
Step 2: Add the Twilio Gem
Include the twilio-ruby gem in your Rails app’s Gemfile:
ruby
gem 'twilio-ruby'
Run bundle install to install the dependency.
If you're working on a legacy Rails application and need help upgrading it to the latest version of Rails, explore our Rails Upgrade services.
Step 3: Configure Twilio Credentials
Store your Twilio credentials securely using environment variables. For development, use the dotenv gem or Rails credentials:
- Create a .env file (add it to .gitignore):
bash
TWILIO_ACCOUNT_SID=your_account_sid
TWILIO_AUTH_TOKEN=your_auth_token
TWILIO_PHONE_NUMBER=+1234567890
- Initialize the Twilio client in config/initializers/twilio.rb:
ruby
Twilio.configure do |config|
config.account_sid = ENV['TWILIO_ACCOUNT_SID']
config.auth_token = ENV['TWILIO_AUTH_TOKEN']
end
Step 4: Create a Twilio Service
Generate a service class to encapsulate SMS logic. Create app/services/twilio_service.rb:
ruby
class TwilioService
def send_sms(to, body)
client = Twilio::REST::Client.new
client.messages.create(
from: ENV['TWILIO_PHONE_NUMBER'],
to: format_phone_number(to),
body: body
)
true
rescue Twilio::REST::TwilioError => e
Rails.logger.error "Twilio Error: #{e.message}"
false
end
private
# Ensure phone numbers are in E.164 format (e.g., +15551234567)
def format_phone_number(number)
number.gsub(/\D/, '').prepend('+')
end
end
Step 5: Integrate with a Controller
Create a MessagesController to handle SMS requests:
ruby
class MessagesController < ApplicationController
def new
# Render a form to collect phone number and message
end
def create
to = params[:phone_number]
body = params[:message]
if TwilioService.new.send_sms(to, body)
redirect_to root_path, notice: 'SMS sent successfully!'
else
redirect_to new_message_path, alert: 'Failed to send SMS.'
end
end
end
Step 6: Create a Form for SMS Input
Add a form in app/views/messages/new.html.erb:
erb
<%= form_with url: messages_path do |f| %>
<div>
<%= f.label :phone_number, "Recipient's Phone Number" %>
<%= f.text_field :phone_number, placeholder: "+1234567890" %>
</div>
<div>
<%= f.label :message, "Message" %>
<%= f.text_area :message %>
</div>
<%= f.submit "Send SMS" %>
<% end %>
Step 7: Test Your Integration
- Start your Rails server: rails s.
- Visit localhost:3000/messages/new and submit a test message.
- If using a Twilio trial account, ensure the recipient’s number is verified.
Step 8: Error Handling & Best Practices
- Validation: Ensure phone numbers are in E.164 format (e.g., +1234567890). Use format_phone_number to sanitize input.
- Rate Limiting: Implement rate limiting to avoid hitting Twilio’s API limits.
- Logging: Log errors for debugging without exposing sensitive data.
- API Failures: Rescue Twilio::REST::TwilioError in the service.
- Async Processing: Avoid blocking web requests by using Active Job.
Step 9: Deploy and Monitor
Deploy your app using platforms like Heroku or Render. Monitor SMS delivery status via the Twilio Console .
Deployment Tips
- Set environment variables in production (e.g., Heroku Config Vars).
- Monitor Twilio logs in the Console for delivery status.
- Consider rate limits and Twilio’s pricing.
- For large-scale apps, hire Rails experts to optimize performance.
Conclusion
With Twilio integration with Rails, you’ve unlocked a powerful tool for user communication. By following this guide, you’ve learned to send SMS messages securely and efficiently. Now, go ahead and enhance your app’s capabilities with Twilio’s expansive features.